Top Python Interview Questions and Answers
Top Python Interview Questions and Answers
What is Python?
What is Python?
Python is a high-level, interpreted programming language known for its readability and simplicity. It supports various programming paradigms, including procedural, object-oriented, and functional programming.
What are the key features of Python?
- Easy to read and write
- Interpreted language
- Dynamically typed
- Extensive standard library
- Community support
What is PEP 8?
PEP 8 is the style guide for Python code, providing conventions on how to write clean and readable code.
What is a Python dictionary?
A dictionary is an unordered collection of key-value pairs. Each key must be unique.
What are lists in Python?
Lists are ordered, mutable collections of items. They can contain mixed data types.
How do you create a function in Python?
Functions are defined using the def keyword, followed by the function name and parameters.
What are Python modules?
Modules are files containing Python code that can define functions, classes, and variables. They can be imported into other modules or scripts.
What is the difference between a list and a tuple?
- List: Mutable, defined with []
- Tuple: Immutable, defined with ()
What is a lambda function?
A lambda function is an anonymous function defined with the lambda keyword. It can take any number of arguments but only has one expression.
What is Generative AI, and how is Python used in its implementation?
 Generative AI involves algorithms that create new data or content based on patterns learned from existing data. Python is often used for implementing these models due to its extensive libraries and frameworks. For instance, TensorFlow and PyTorch are popular Python libraries for building and training generative models like GANs (Generative Adversarial Networks) and VAEs (Variational Autoencoders).
What are some Python libraries that support Generative AI, and what are their uses?
Key Python libraries for Generative AI include:
- TensorFlow: Provides tools for building and training deep learning models, including generative models.
- PyTorch: Offers a flexible and dynamic approach to building and training models, popular for GANs and other generative models.
- Keras: A high-level API that runs on top of TensorFlow, making it easier to build and train neural networks, including generative models.
What is Prompt Engineering, and how can Python be used to facilitate it?
A4: Prompt Engineering involves designing input prompts to guide AI models in generating specific outputs. Python can facilitate this by using libraries and APIs that interact with generative models. For example, the OpenAI Python client library can be used to send prompts to models like GPT-3 and receive generated responses, allowing for experimentation with different prompt designs.
How can Python be used to test and refine prompts for generative models?
Python can be used to automate the testing and refinement of prompts by:
- Scripting Prompts: Writing scripts that send various prompts to a generative model and collect responses.
- Analyzing Results: Using Python libraries like pandas for analyzing the quality and relevance of generated outputs.
- Iterative Testing: Automating the process of testing different prompt variations and evaluating their effectiveness.
How can Python be used to monitor machine learning models in production?
 Python can be used for monitoring models through:
- Metrics Collection: Using libraries like
Prometheus_client
to expose metrics that can be collected and monitored. - Visualization: Integrating with visualization tools like Grafana to create dashboards for real-time monitoring.
- Alerting: Writing Python scripts or applications that trigger alerts based on model performance or anomalies.
 What are some Python-based tools or frameworks commonly used in MLOps for model deployment and management?
 Common Python-based tools for MLOps include:
- Docker: For containerizing Python applications and models.
- Flask or FastAPI: For creating APIs to serve machine learning models.
- TensorFlow Serving or TorchServe: For serving models built with TensorFlow or PyTorch.
- MLflow: For tracking experiments, managing models, and deploying them in Python.
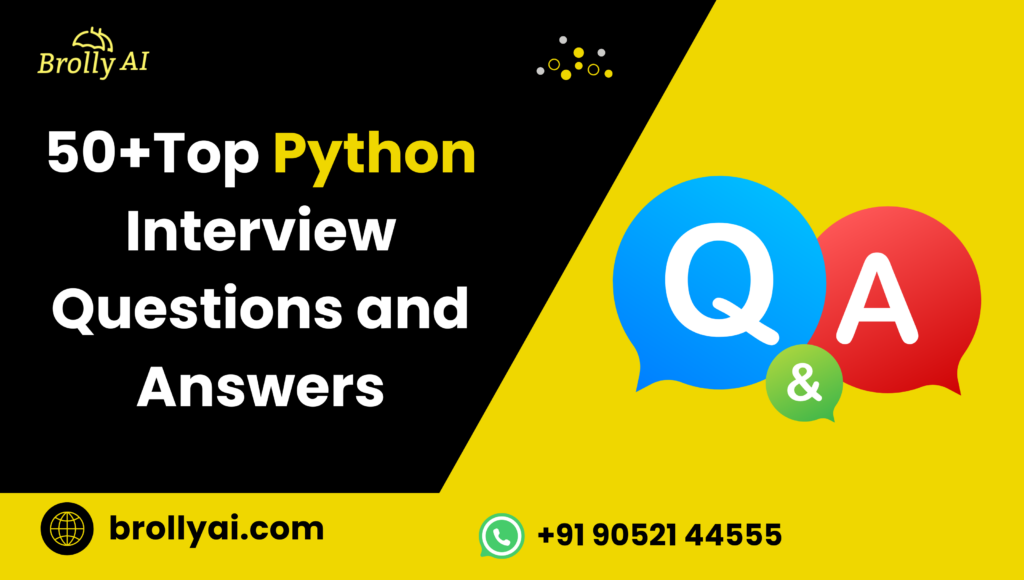
What is the use of the self keyword?
self represents the instance of the class and is used to access attributes and methods associated with the current instance.
What is slicing in Python?
Slicing is a way to extract a portion of a sequence, like a list, string, or tuple.
my_list = [1, 2, 3, 4]
print(my_list[1:3]) # Output: [2, 3]
@decorator_name
syntax. What are Python comprehensions?
Comprehensions provide a concise way to create lists, dictionaries, and sets. They consist of an expression and a for-loop.
squares = [x**2 for x in range(10)]
What is the purpose of the pass
statement?
The pass
statement does nothing and is used as a placeholder for future code.
Exceptions are errors detected during execution. They can be caught and handled using
try
and except
blocks. How do you handle exceptions in Python?
Exceptions are handled using try, except, else,
and
finally
blocks.
range
and xrange
in Python 2.x? range
returns a list, while xrange
returns an iterator. In Python 3.x, range
behaves like xrange
. Generators are iterators that yield items one at a time using theÂ
yield
keyword. They are memory efficient. What are Python iterators?
An iterator is an object that contains a countable number of values and can be iterated over. It implements the __iter__() and __next__() methods.
What is the purpose of the with statement?
The with statement is used to wrap the execution of a block of code with methods defined by a context manager, ensuring proper acquisition and release of resources.
What is the difference between == and is in Python?
== checks for value equality, while is checks for object identity (whether two references point to the same object).
What are Python’s built-in data types?
- Numeric: int, float, complex
- Sequence: list, tuple, range
- Text: str
- Mapping: dict
- Set: set, frozenset
- Boolean: bool
What is __init__ in Python?
__init__ is a special method in Python classes, known as a constructor. It is automatically called when an instance of the class is created.
What is the difference between deep copy and shallow copy?
- Shallow copy: Creates a new object, but inserts references into it to the objects found in the original.
- Deep copy: Creates a new object and recursively copies all objects found in the original.
What are *args and **kwargs?
- *args allows a function to accept any number of positional arguments.
- **kwargs allows a function to accept any number of keyword arguments.
What is a Python set?
A set is an unordered collection of unique items.
What is the len() function?
len() returns the number of items in an object.
What are Python strings?
Strings are sequences of characters enclosed in quotes (single, double, or triple).
How do you convert a string to a list in Python?
You can convert a string to a list using the list() function or split() method.
my_string = "Hello"
my_list = list(my_string) # Output: ['H', 'e', 'l', 'l', 'o']
What is the __repr__() method?
__repr__() is used to define the official string representation of an object, useful for debugging.
What is __str__() method?
__str__() is used to define the informal string representation of an object, suitable for end users.
What are Python classes?
Classes are blueprints for creating objects. They encapsulate data for the object and methods to manipulate that data.
What is inheritance in Python?
Inheritance allows a class to inherit attributes and methods from another class, promoting code reuse.
What is a superclass and a subclass?
- Superclass: The class from which attributes and methods are inherited.
- Subclass: The class that inherits attributes and methods from the superclass.
What is method overriding?
Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass.
What is method overloading?
Python does not support method overloading directly. You can achieve similar behavior by using default arguments.
What is a Python package?
A package is a collection of related modules organized in a directory hierarchy.
What is the purpose of the __init__.py file?
The __init__.py file is used to mark a directory as a Python package and can also be used to execute initialization code.
What are Python’s built-in functions?
Python has numerous built-in functions like print(), len(), type(), int(), float(), and many more.
What is the purpose of the dir() function?
dir() returns a list of valid attributes for an object.
What is the difference between remove(), del, and pop() in lists?
- remove(): Removes the first occurrence of a value.
- del: Deletes an item or slice.
pop(): Removes and returns an item at a given index.
What are docstrings in Python?
Docstrings are string literals that appear right after the definition of a function, method, class, or module, used to document what the function or class does.
How do you comment in Python?
Single-line comments use the # symbol. Multi-line comments can use triple quotes ”’ or “””.
What is a virtual environment in Python?
A virtual environment is an isolated environment that allows you to install packages and dependencies specific to a project, without affecting the global Python installation.
What is the map() function?
map() applies a given function to all items in an iterable and returns a map object (iterator).
What is the filter() function?
filter() constructs an iterator from elements of an iterable for which a function returns true.
What is reduce() function?
reduce() applies a rolling computation to sequential pairs of values in an iterable and returns a single value.
What is list comprehension?
List comprehension provides a concise way to create lists using an expression followed by a for-loop inside square brackets.
What is the join() method in strings?
The join() method concatenates a list of strings into a single string with a specified separator.
','.join(['a', 'b', 'c']) # Output: 'a,b,c'
What is the split() method in strings?
The split() method breaks a string into a list of substrings based on a specified delimiter.
What is a binary search?
Binary search is an efficient algorithm for finding an item in a sorted list by repeatedly dividing the search interval in half.
What is the Global Interpreter Lock (GIL)?
The GIL is a mutex that protects access to Python objects, preventing multiple native threads from executing Python bytecodes simultaneously.
What is a Python set comprehension?
Set comprehension creates a set based on an existing iterable using a concise syntax.
{x**2 for x in range(10)} # Output: {0, 1, 4, 9, 16, 25, 36, 49, 64, 81}
What is a try-except block?
A try-except block is used to catch and handle exceptions. Code that might raise an exception is placed in the try block, and the handling code is in the except block.
What is a finally block?
The finally block executes code regardless of whether an exception occurred in the try block or not, typically used for cleanup actions.
What is the difference between assert and raise?
- assert: Used for debugging purposes to check if a condition is true.
- raise: Used to raise an exception manually.
What is a Python class method?
A class method is a method that is bound to the class and not the instance. It can be called on the class itself. It is defined using the @classmethod decorator and takes cls as the first parameter.
What is a static method in Python?
A static method is a method that does not access or modify the class state. It is defined using the @staticmethod decorator and does not take self or cls as the first parameter.
What is monkey patching in Python?
Monkey patching refers to dynamically modifying or extending a module or class at runtime.
What are Python’s built-in modules?
Python has many built-in modules like math, sys, os, datetime, json, and more, providing various functions and utilities.
Upgrading Software Engineers to Generative AI Engineers Brolly AI is your trusted partner for top-quality training courses. Our commitment to excellence ensures that you receive the most comprehensive and up-to-date education in your chosen field. With a focus on practical skills and industry relevance, our courses are designed to empower you with the knowledge and expertise needed to succeed. Join Brolly AI for a trans-formative learning experience that propels your career to new heights. Quality training, tailored for your success